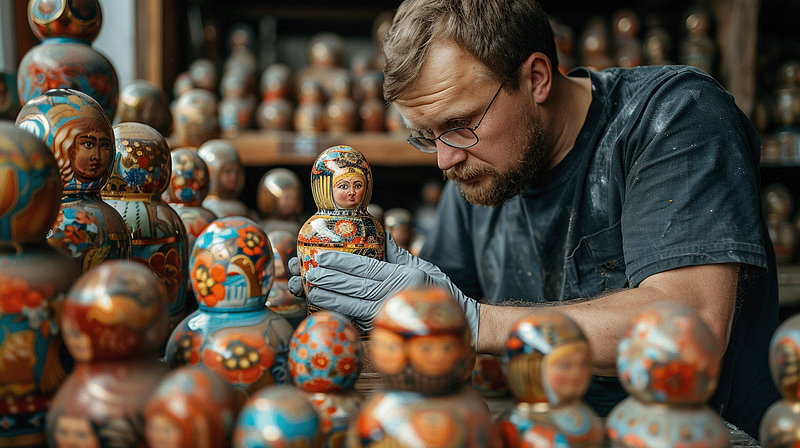
Caching So Good, It’s Like IKEA Furniture You Built Correctly
Your no-BS weekly brief on software engineering.
Join 100,000+ developers
If there are three hard problems in computer science, they are, without question: naming things, caching, and off-by-one errors. While I can’t help you debug your loop indexing woes here, I can dive into caching—specifically, Russian doll caching—to help demystify this powerful yet sometimes misunderstood technique. Buckle up; it’s going to be an oddly satisfying ride, like assembling IKEA furniture correctly on the first try.
What Is Russian Doll Caching?
Russian doll caching is a technique where cached elements are nested within each other, similar to the structure of traditional Russian nesting dolls. This approach is particularly useful in scenarios where a parent object’s cache can be invalidated without affecting the caches of its child components. It’s like giving your data a carefully organized backpack: each item has its own pocket, so when you need to swap out a snack (or update a comment), you don’t have to dump out the entire bag.
Efficiency meets organization.
For example, imagine a blog platform. Each post has multiple comments, and those comments might have user avatars or likes attached to them. With Russian doll caching, the post can cache the overall structure while each comment (and its metadata) is cached individually. This means if a user updates their avatar, you don’t need to regenerate the entire post’s cache—just the relevant pieces. It’s like only washing the dishes you actually used—efficiency at its finest.
Why Use Russian Doll Caching?
Strengths:
- Granularity: You can invalidate smaller pieces of the cache without disrupting larger structures. Think of it like replacing a squeaky wheel instead of buying a whole new car.
- Performance: By caching at multiple levels, you reduce the computational overhead of regenerating entire pages or structures.
- Maintainability: If your data model changes, you can update the caching logic incrementally rather than reworking a monolithic cache structure.
Weaknesses:
- Complexity: Nesting caches can introduce bugs, especially if you’re not meticulous about managing cache keys and dependencies. It’s a bit like trying to assemble IKEA furniture without the instructions—risky at best.
- Invalidation Overhead: Keeping track of what needs to be invalidated and when can be a headache. Forgetting to invalidate a cache is the developer equivalent of leaving milk in the fridge too long—everything stinks eventually.
- Cache Contention: Nested caches increase the number of potential keys in use, which can strain your caching infrastructure.
When to Use Caching—and When Not To
Before jumping into caching strategies, ask yourself: do I even need to cache this? Over-caching can lead to maintenance nightmares and bugs. As a rule of thumb:
Cache when:
- Your application frequently renders the same data.
- The data is expensive to compute or retrieve.
- There are no high-frequency updates to the underlying data.
Don’t cache when:
- The data is volatile, with frequent updates.
- The computational cost of generating the data is low.
- You’re in the early stages of development (build the feature first, optimize later). Trust me, you don’t need a cache for your Hello World app.
Russian Doll Caching: A Server-Side Rendering Solution
Russian doll caching is primarily a server-side rendering (SSR) solution, where fragments of the response—such as a page’s header, main content, or comments—are cached separately. This approach works particularly well for SSR frameworks like Rails, Django, or even Node.js-based SSR tools. Here’s why:
- Fragment Caching for Views: In SSR, a page is typically composed of multiple fragments (e.g., headers, footers, articles). By caching these fragments individually, servers can serve partially cached responses, improving performance while keeping dynamic parts updated.
- Reduced Latency: Since SSR often has higher latency compared to client-rendered applications, fragment caching can mitigate this by serving pre-rendered components quickly.
- Optimized Bandwidth: Nested caching ensures only the changed parts of a page are recomputed or re-fetched, reducing both computational overhead and bandwidth usage.
While the principles can extend to client-side frameworks like React, SSR environments benefit the most because they often handle the full rendering pipeline. In React, similar concepts might apply in server-side setups, such as with Next.js or frameworks that integrate hydration strategies for cached SSR pages.
Implementing Russian Doll Caching: Framework-Agnostic Principles
Russian doll caching is not unique to Rails or any specific framework. The underlying principles can be applied across different languages and platforms:
- Fragment Caching: Break down your views or responses into smaller components and cache each independently. This ensures that updates to one fragment don’t invalidate the entire response.
- Cache Keys: Use unique and predictable keys for each fragment. Incorporate versioning or timestamps to ensure that updates invalidate the appropriate cache.
- Invalidation Strategy: Define clear rules for when and how caches should be invalidated. For example, changes to a parent object might trigger cache invalidation for child components, but the reverse might not always hold true.
- Monitoring and Debugging: Implement tools to monitor cache performance and debug key usage. Cache hit rates and invalidation patterns are critical metrics.
Example in Practice:
Consider a blogging platform. Each post might have:
- A cached list of comments.
- Individual caches for each comment.
When a new comment is added, only the comment list and the specific comment cache should be invalidated. The post body and other cached fragments remain untouched. It’s like upgrading the tires on your car without having to replace the entire engine.
Russian Doll Caching in Rails 8 (or Beyond)
Rails provides excellent support for implementing Russian doll caching, but these principles apply broadly:
Fragment Caching:
<% cache @post do %>
<%= @post.title %>
<%= @post.body %>
<% @post.comments.each do |comment| %>
<% cache [@post, comment] do %>
Cache Key Management
Ensure cache keys are tied to the objects’ state. For example, in a non-Rails framework, you might compute keys like this:
# Example in Python
cache_key = f"comment:{comment.id}:{comment.updated_at.timestamp()}"
This approach works regardless of language or framework, ensuring caches are invalidated when the object’s state changes.
Cache Store:
Select a cache store that suits your application’s needs, whether it’s Redis, Memcached, an in-memory solution, or even SQLite—especially since Rails 8 now highlights SQLite as a lightweight but powerful option for caching data.
Common Pitfalls of Russian Doll Caching
Over-invalidation: Broad cache keys can invalidate too much data unnecessarily. Use scoped keys to target specific fragments.
Stale Data: Without proper key versioning, outdated data can persist. Always include timestamps or version numbers.
Complexity: Keep caching logic simple. Overly complex caching layers can become harder to debug than the problems they aim to solve. It’s like adding duct tape to fix a leaky pipe—temporary at best.
A Brief Note on Naming and Cache Invalidation
If you’ve ever been burned by a cache bug, you know that naming your keys well and managing invalidation are some of the hardest parts of this process. Poorly scoped keys can lead to hard-to-reproduce errors, while overly aggressive invalidation can reduce performance gains.
So is this my new hammer for performance problems?
Russian doll caching is an elegant solution to a common problem, but it’s not a silver bullet. The principles of fragment caching, key management, and invalidation strategies are applicable across frameworks and languages. Use it where it makes sense, and don’t let the allure of clever caching distract you from the fundamentals of good design.
And if you’re stuck debugging a caching issue at 2 AM, just remember: it’s not you, it’s the cache (probably).
<%= comment.body %>
<%= comment.user.name %>